NSUserDefaults
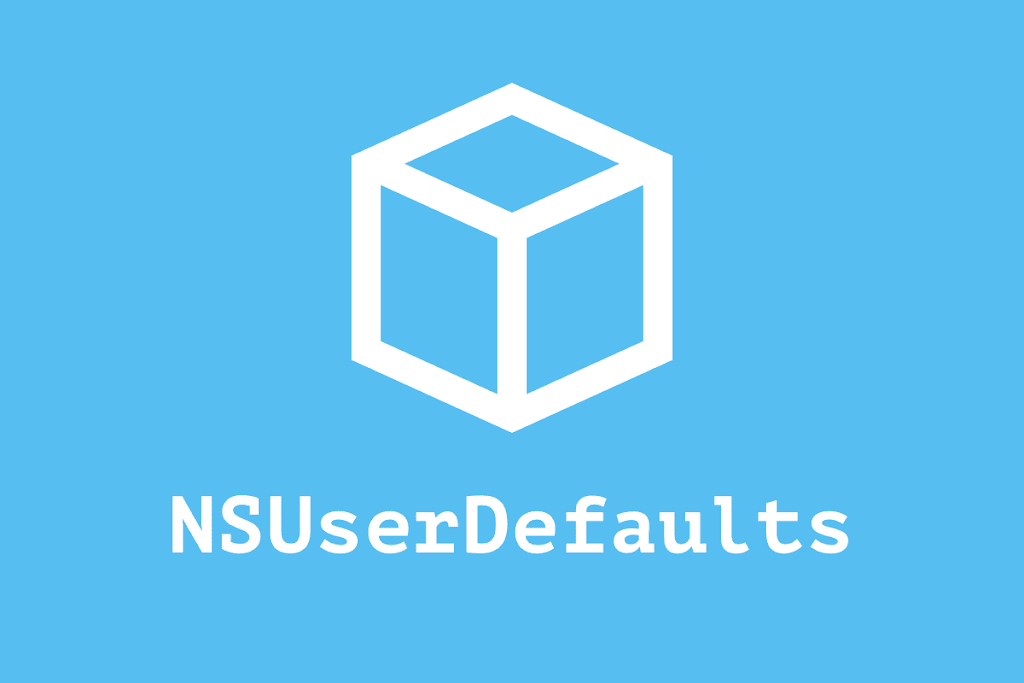
The NSUserDefault
class is a helpful class for saving defaults and user settings that persist for as long as app is installed. The data is stored and retrieved with a key-value pair mechanism. The reason the data stored by the NSUserDefaults
class is called defaults is because this class is typically used to store default application state that gets loaded when an app launches. The defaults interface should be used for small key-value pairs such as settings. and not be misused to store larger sets of data, as that will slow down your app at launch time.
To speed up access to the values stored in the defaults database, NSUserDefaults
caches the data in an in-memory cache, which avoids having to reopen the database on every read access. To keep this in-memory cache up to date and synchronized with the defaults database, NSUserDefaults
periodically calls the synchronize()
method. Since synchronize()
is already being called periodically by the application to keep the in-memory and the defaults database in synch, there is no need to manually call synchronize()
anymore. You should only call synchronize()
manually if for some (very good) reason you cannot wait for synchronize()
to be called automatically by the application. This is what apple has to say about calling the synchronize()
method.
Because this method is automatically invoked at periodic intervals, use this method only if you cannot wait for the automatic synchronization (for example, if your application is about to exit) or if you want to update the user defaults to what is on disk even though you have not made any changes.
Types stored in NSUserDefaults
Any .plist type can be stored by NSUserDefaults
. These types are NSString(String)
, NSArray(Array)
, NSDictionary(Dictionary)
(for both NSArray
and NSDictionary
types their contents must be property list objects), NSNumber(Int, Float, Double, Boolean)
, NSDate
and NSData
. The types in parentheses are the corresponding types in Swift. If you want to store data of some arbitrary type with NSUserDefaults you can always do so by creating an instance of NSData and archiving your data in it. However, remember to not misuse NSUserDefaults for data storage for large amounts of data.
Note that all objects that you save in the defaults database with NSUserDefaults are immutable when you retrieve them at a later point. This is true even if you used a mutable object when storing the value in the first place.
Working with NSUserDefaults
To get an instance of the shared defaults object simply call the standardUserDefaults() class method on NSUserDefaults
let defaults = NSUserDefaults.standardUserDefaults()
Setting defaults values is as simple as calling the appropriate set method for the data type to be saved. For an integer default, for instance, you would call
defaults.setInteger(myInt, forKey: myIntegerDefaultKey)
Similar methods exist for Boolean
, Double
, Float
, URL
and Object
(for NSData
, NSString
, NSArray
, NSDictionary
, NSDate
, NSDate
and NSNumber
)
To find out whether a user default with a certain name already exists you can use the objectForKey()
method. This method takes a String key as its only parameter and returns an optional AnyObject
. If the return value is nil
, it means that no such default exists.
For the other types, similar methods to objectForKey()
also exist for retrieving defaults values. Depending on whether the key exists, these methods either return the stored value or some default value for when the key does not exist.